Solidity Programming Language: Guide for Beginner Developers
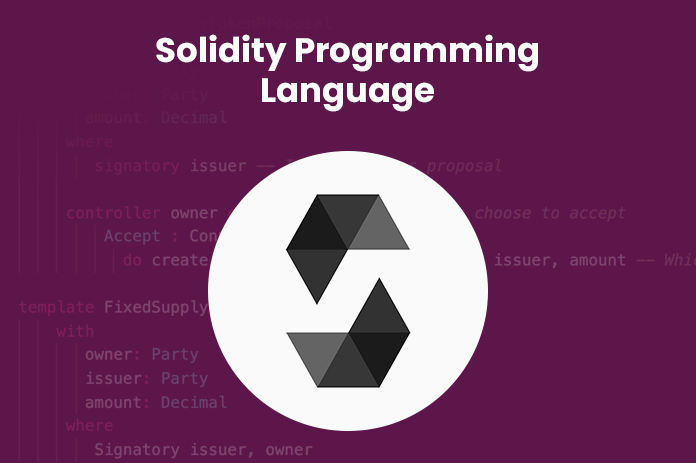
There’s no denying the importance of Solidity in the world of blockchain development. As the core programming language for writing smart contracts on the Ethereum platform, Solidity plays a crucial role in creating decentralized applications and executing code on the blockchain.
In this blog post, we will discuss the definition of Solidity, explore its various use cases, and examine into the concept of variables within Solidity programming.
Let’s get started…
What is Solidity?
Solidity is an advanced programming language tailored specifically for the creation of smart contracts on the Ethereum blockchain. It is statically typed, supports inheritance, and provides various other features that make it suitable for developing decentralized applications (dApps) and other blockchain-based solutions.
Solidity was first introduced in 2014 by Gavin Wood, one of the co-founders of Ethereum, and has since become the most widely used language for writing smart contracts.
Solidity enables developers to intricately craft the regulatory framework and operational logic of their applications with utmost security and transparency.
With Solidity, developers have the ability to develop self-executing contracts that uphold the terms and conditions embedded within them automatically. These contracts are stored on the blockchain and anyone can access and execute with the necessary permissions. It eliminates intermediaries and ensures that transactions are executed exactly as intended.
For instance, Solidity includes data types like uint (unsigned integer) and address for handling numerical values and Ethereum addresses, respectively.
Besides it, Solidity supports complex data structures as well. Such as arrays and mappings, along with functions for interacting with other contracts on the blockchain.
Syntax and Structure of Solidity
The syntax of Solidity is similar to that of JavaScript (JS), making it very easy for developers familiar with JavaScript or other C-like languages to learn. Solidity code is structured into contracts, which are similar to classes in object-oriented programming. Each contract can contain variables, functions, and modifiers, which define the behavior of the contract.
Comments and whitespace are also important aspects of Solidity code. Single-line comments start with //, while multi-line comments are enclosed within /* */. Whitespace, such as spaces, tabs, and line breaks, is generally ignored by the compiler but can be used to improve code readability.
Below are the key aspects of Solidity’s syntax and structure to provide a solid foundation for building reliable decentralized applications:
- Data Types: Solidity offers a variety of data types including integers, booleans, strings, arrays, and structs. Developers should be familiar with these data types and how to declare and use them effectively in their smart contracts.
- Functions: Functions in Solidity are similar to functions in other programming languages, with the added ability to specify visibility (public, private, internal, external) and modifiers. Understanding how to define and call functions is crucial for defining the behavior of smart contracts.
- Control Structures: Solidity includes common control structures like if statements, for loops, while loops, and switch cases. These structures allow developers to control the flow of their smart contracts and implement complex logic.
- Modifiers: Modifiers in Solidity are special functions able to modify the behavior of other functions. They are often utilized to add access control or require certain conditions to be met before executing a function.
- Events: Events in Solidity are used to communicate information to the outside world, typically for logging or debugging purposes. Understanding how to define and emit events is essential for building transparent and auditable smart contracts.
- Inheritance: Solidity supports inheritance, allowing smart contracts to inherit properties and methods from other contracts. By leveraging inheritance, developers can create modular and reusable code, saving time and effort in the development process.
- Error Handling: Proper error handling is crucial in smart contract development to prevent vulnerabilities and ensure the robustness of the code. Solidity provides mechanisms such as assert, require, revert, and throw to handle exceptional conditions and errors effectively.
Example of Simple Contract Written in Solidity Language
pragma solidity ^0.5.16;
contract SimpleContract {
//state variable
uint public count;
//function
function incrementCount() public {
count += 1;
}
}
In this contract, pragma solidity ^0.5.16; indicates the version of Solidity being used. The contract SimpleContract contains a state variable count and a function incrementCount() which increases the value of count by one.
8 Advantages of Using Solidity for Smart Contract Development
Below are the eight key benefits of using Solidity for smart contract development:
- Ethereum Compatibility: Solidity is the official language for writing smart contracts on the Ethereum platform. This compatibility opens up a world of opportunities for decentralize applications (DApps) and token issuance.
- Security and Transparency: Solidity allows developers to write secure and transparent smart contracts by providing features such as strong typing, visibility specifiers, modifiers, exception handling, and access control mechanisms. This helps prevent common vulnerabilities such as reentrancy attacks, integer overflows, and unauthorized access to contract functions and data.
- Community Support: Solidity benefits from a very engaging and active community of blockchain developers who contribute to its evolution and provide resources for learning and troubleshooting. This support network is invaluable for developers seeking guidance and collaboration in their smart contract projects.
- Ease of Learning: Solidity features a syntax similar to widely-used programming languages like JavaScript, making it accessible to developers with programming experience in other languages. The Ethereum developer documentation offers comprehensive guides and tutorials to aid in the learning process.
- Versatility: Solidity supports a wide range of use cases beyond simple token creation, including complex smart contracts with multiple functions and logic. Developers can leverage Solidity’s flexibility to tailor smart contracts to suit specific requirements and business needs.
- Interoperability: Solidity enables interoperability between different smart contracts and decentralized applications on the Ethereum blockchain, fostering a cohesive and interconnected ecosystem. This interoperability is essential for the seamless exchange of assets and information on blockchain networks.
- Efficiency and Cost-effectiveness: Solidity is designed to be efficient and cost-effective in terms of gas usage. Gas is the unit of measurement for computational effort required to execute operations on the Ethereum blockchain. By optimizing their Solidity code, developers can reduce gas costs and improve an efficiency of their smart contracts.
- Decentralization and Immutability: dApps created using Solidity programing language are not controlled by any central authority and are resistant to censorship and tampering. Once deployed, Solidity smart contracts are immutable, meaning that their code and state cannot be modified or deleted.
Use Cases of Solidity
Solidity, as the main programming language for Ethereum smart contracts, has a wide range of use cases.
Let’s explore some of the primary use cases for Solidity.
Decentralized Finance (DeFi)
Solidity plays a crucial role in the explosive growth of DeFi applications. Smart contracts written in Solidity power various DeFi protocols such as decentralized exchanges (DEXs), lending platforms, yield farming, and more. These smart contracts enable users to interact with financial services in a trustless manner, without the need for intermediaries.
Non-Fungible Tokens (NFTs)
NFTs have revolutionized the digital art, gaming, and collectibles industries. Solidity is used to create NFT smart contracts that define the unique characteristics and ownership rights of digital assets. Artists, game developers, and collectors leverage Solidity to mint, trade, and manage NFTs securely on blockchain networks.
Governance and Voting Systems
Solidity enables the creation of transparent and secure governance and voting systems. By deploying smart contracts written in Solidity, organizations can conduct fair elections, stakeholder voting, and decision-making processes on the blockchain. This ensures verifiability, immutability, and resistance to tampering in the voting mechanisms.
Supply Chain Management
Blockchain technology combined with Solidity can streamline supply chain processes by creating transparent and traceable systems. Smart contracts can be used to automate inventory tracking, product provenance verification, and payment settlements between stakeholders. This enhances efficiency, reduces fraud, and increases trust among supply chain participants.
Gaming and Decentralized Applications (DApps)
Solidity is utilized in creating decentralized applications and games that run on blockchain networks. By coding logic into smart contracts, developers can implement in-game assets, rules, and interactions in a secure and decentralized manner. Gamers and users benefit from enhanced ownership, interoperability, and fairness in decentralized gaming ecosystems.
Developing Decentralized Autonomous Organizations (DAOs)
A DAO is an entity that operates based on rules encoded within a computer program. It functions in a transparent manner, controlled by its members, and remains independent of any central authorities.
DAOs utilize blockchain technology for decision-making and internal organization through the use of tokens that grant voting rights. These organizations are essentially intricate smart contracts where the rules and regulations of the decentralized entity are intricately woven into the code using Solidity.
Implementing Initial Coin Offerings (ICOs)
Solidity has also been used to write the smart contracts needed to carry out Initial Coin Offerings (ICOs). An Initial Coin Offering commonly used by cryptocurrencies as a crowdfunding method offers a portion of the cryptocurrency being crowdfunded to investors via “tokens” in exchange for legal tender or other cryptocurrencies.
In brief, Solidity serves as a powerful tool for implementing complex business logic, automating processes, and enabling innovative solutions on the blockchain. Its versatility and robust features make it a suitable choice for developers looking to develop decentralized applications across various industries.
Key Features of Solidity Programming Language
Solidity has several key features that make it a powerful language for writing smart contracts:
- Contract-oriented Programming: Solidity is a contract-oriented programming language, meaning that it focuses on defining contracts that encapsulate the behavior and state of a system. Contracts can interact with each other through function calls and events.
- Built-in Data Structures: Solidity provides built-in data structures such as arrays, mappings, and structs, which allow developers to store and manipulate data efficiently.
- Function Modifiers: Solidity allows developers to define function modifiers, which are used to modify the behavior of functions. Modifiers can be used to add access control, validate inputs, or perform other operations before or after a function is executed.
- Events and Logging: Solidity supports events, which are used to notify external applications about specific occurrences within a smart contract. Events can be logged and subscribed to by external applications, allowing them to react to changes in the contract’s state.
- Library Support: Solidity provides support for importing external libraries, enabling developers to reuse code and integrate existing solutions seamlessly into their contracts. This feature promotes modularity and streamlines the development process.
- Contract Inheritance: Inheritance in Solidity enables developers to create new contracts based on existing ones, promoting code reusability and scalability. This feature simplifies contract development by allowing for the extension and modification of contract functionality.
Solidity Data Types and Variables
Solidity offers a range of data types and variables that play a key role in defining how data is stored and manipulated within contracts. In Solidity, data types can be classified into two different categories: value types and reference types.
1. Value Types in Solidity
Value types in Solidity are data types that hold a specific value directly. These types are passed by value, meaning when they are assigned to a variable or passed as a function argument, a copy of the data is created.
- Boolean:Â The boolean type in Solidity represents true or false values. It is often used for conditions and control flow.
- Integers and Unsigned Integers:Â Solidity supports various sizes of integers, both signed (int) and unsigned (uint). The size of the integer determines the range of values it can hold.
- Addresses:Â Addresses in Solidity are used to store Ethereum addresses. They are 20 bytes in length and can represent smart contracts or external accounts.
- Fixed Point Numbers:Â Solidity provides fixed point numbers, which are useful for representing decimal numbers with a fixed number of decimal places.
2. Reference Types in Solidity
Reference types in Solidity store references to data rather than the data itself. These types can represent more complex data structures and are passed by reference, meaning they refer to the original data.
- Arrays:Â Arrays in Solidity can store multiple values of the same type sequentially. They can be dynamically sized or fixed.
- Structs:Â Structs allow developers to define custom data structures that can hold multiple variables of different types together.
- Mappings:Â Mappings are key-value data structures that are often used to store data in a key-value pair fashion.
- Strings:Â Solidity supports strings, which are arrays of UTF-8 encoded bytes. Strings are reference types and have dynamic size.
3. Special Data Types
- Contract Types: A contract defined in Solidity can be used as a type. A contract type variable holds the address where the contract resides.
- Function Types: Solidity supports function types as first-class types. Function types variables can be assigned from functions and called.
By understanding the different data types in Solidity and how they are used, developers can create efficient and secure smart contracts that interact with the Ethereum blockchain.
Control Structures and Functions in Solidity
Solidity provides various control structures and functions for implementing conditional logic and loops:
- Conditional Statements: Solidity supports if-else statements for implementing conditional logic based on boolean expressions. It also supports switch statements for handling multiple possible values of a variable.
- Loops: Solidity provides for loops, while loops, and do-while loops for implementing iterative logic. Such loops allow developers to execute a specific code segment repeatedly until a predefined condition gets satisfied.
- Functions and Function Overloading: Solidity allows developers to define functions that can be called from other contracts or externally.
- Function Visibility and Modifiers: Solidity provides different visibility modifiers for functions, such as public, private, internal, and external. These modifiers control the accessibility of functions within a contract and how they can be called.
Object-Oriented Programming in Solidity
Solidity supports object-oriented programming (OOP) concepts such as classes, objects, inheritance, and polymorphism:
- Classes and Objects: Solidity allows developers to define classes, which act as blueprints for creating objects.
- Inheritance and Polymorphism: Solidity supports single inheritance, meaning that a contract can inherit from only one parent contract. Inheritance allows developers to reuse code and create more specialized contracts based on existing ones. Polymorphism is also supported, allowing contracts to be treated as instances of their parent contracts.
- Abstract Contracts and Interfaces: Solidity allows the creation of abstract contracts, which cannot be instantiated but can be used as base contracts for other contracts. Interfaces stands for a set of function signatures that a contract must implement, allowing for interoperability between different contracts.
Debugging and Testing Solidity Code
Debugging and testing are important aspects of Solidity development to ensure the correctness and reliability of smart contracts:
- Debugging Tools and Techniques: Solidity provides various debugging tools and techniques, such as using console.log statements or event logging to track the execution flow of a contract. External tools like Remix IDE also provide debugging capabilities.
- Unit Testing Frameworks: Solidity has several unit testing frameworks available, such as Truffle and Hardhat. Such frameworks help developers to write automated tests to verify the behavior of their contracts and catch any potential bugs or vulnerabilities.
- Integration Testing and Deployment Testing: In addition to unit testing, it is important to perform integration testing to ensure that different components of a dApp work together correctly. Deployment testing involves testing the deployment process of smart contracts to ensure that they are deployed correctly and function as expected.
FAQs about Solidity Programing Language
Is Solidity Harder than Python?
Yes, Solidity is harder than Python. While both Solidity and Python are object-oriented, Solidity is considered more difficult due to its focus on blockchain applications. However, it offers more features for complex contracts compared to Python.
Is it Hard to Learn Solidity Programing Language?
Learning Solidity programming language can be challenging due to its unique syntax and blockchain-specific concepts. However, with dedication and practice, mastering Solidity is achievable within 1-6 months.
Is Solidity Still Worth Learning?
Yes, learning Solidity is still worth it. As the programming language for Ethereum smart contracts, it offers high demand for blockchain developers and opportunities in decentralized finance (DeFi) and NFTs. Mastering Solidity can lead to lucrative career options in the growing blockchain industry.
How Long will it Take to Learn Solidity?
Learning Solidity programing language for Ethereum smart contracts, may take about 1-6 months for basic proficiency. Mastery can take 1-2 years, depending on prior experience and dedication to learning.
How much do Solidity Developers Make?
Solidity developers make an average salary of around $120,000 per year. Salaries of solidity programmers can range from $80,000 to $160,000 based on experience, location, and company size.
Can you Learn Solidity with no Coding Experience?
Yes, you can learn Solidity with no coding experience as basic Solidity programming is easy to learn through effective tutorial tactics for beginners.
To wrap up
In brief, with simple and familiar syntax, Solidity helps developers to create secure and efficient decentralized applications effortlessly.
Understanding the different data types and variables in Solidity is crucial for building robust smart contracts that can handle complex use cases.
By grasping the above discussed basics of Solidity programing language, you can be an expert solidity developer and can unlock the full potential of blockchain technology and create innovative decentralized solutions.